#include <Wire.h>
int PowerHead1 = 39;
int PowerHead2 = 41;
int PowerHead3 = 43;
int PowerHead4 = 45;
int resFan = 47;
int powerHead = 49;
byte PowerHead1State = LOW;
byte PowerHead2State = LOW;
byte PowerHead3State = LOW;
byte PowerHead4State = LOW;
byte ResFanState = LOW;
byte PowerHeadState = LOW;
// THESE CONSTANTS SET THE TIMING AND FUNCTIONALITY *******************************
/* 10 seconds is 10000
* 1 minute is 60000
* 5 minutes is 300000
* 10 minutes is 600000
* 1 hour is 3,600,000
* 6 hours is 21,600,000
* 18 hours is 64,800,000
*
* Do not exceed 32000 unless you change the ints to longs
*/
const double PowerHead1Interval = 480000;
const double PowerHead2Interval = 480000;
const double PowerHead3Interval = 480000;
const double PowerHead4Interval = 480000;
const int ResFanInterval = 1000;
const int PowerHeadInterval = 32000; // bottom left plug
const double PowerHead1Duration = 120000;
const double PowerHead2Duration = 120000;
const double PowerHead3Duration = 120000;
const double PowerHead4Duration = 120000;
const int ResFanDuration = 12000;
const int PowerHeadDuration = 3000;
//*********************************************************************************
unsigned long currentMillis = 0; // stores the value of millis() in each iteration of loop()
unsigned long previousPowerHead1Millis = 0;
unsigned long previousPowerHead2Millis = 0;
unsigned long previousPowerHead3Millis = 0;
unsigned long previousPowerHead4Millis = 0;
unsigned long previousResFanMillis = 0;
unsigned long previousPowerHeadMillis = 0;
void setup() {
Serial.begin(9600);
Serial.println("Starting RedJay FogPonics Grow System");
Wire.begin();
pinMode(PowerHead1, OUTPUT);
pinMode(resFan, OUTPUT);
pinMode(PowerHead2, OUTPUT);
pinMode(PowerHead3, OUTPUT);
pinMode(PowerHead4, OUTPUT);
pinMode(powerHead, OUTPUT);
pinMode(51, OUTPUT); //Not Used
pinMode(53, OUTPUT); //Not Used
digitalWrite(PowerHead1, HIGH); // HIGH is off
digitalWrite(resFan, HIGH);
digitalWrite(PowerHead2, HIGH);
digitalWrite(PowerHead3, HIGH);
digitalWrite(PowerHead4, HIGH);
digitalWrite(powerHead, HIGH);
digitalWrite(51, HIGH);
digitalWrite(53, HIGH);
}
void loop() {
currentMillis = millis();
updatePowerHead1State();
updatePowerHead2State();
updatePowerHead3State();
updatePowerHead4State();
updateResFanState();
updatePowerHeadState();
}
void updatePowerHead1State() {
if (PowerHead1State == LOW) {
// PowerHead1State = HIGH;
// digitalWrite(PowerHead1, LOW);
// }
if (currentMillis - previousPowerHead1Millis >= PowerHead1Interval) {
// time is up, so change the state to HIGH
PowerHead1State = HIGH;
digitalWrite(PowerHead1, LOW);
// and save the time when we made the change
previousPowerHead1Millis += PowerHead1Interval;
}
}
else {
if (currentMillis - previousPowerHead1Millis >= PowerHead1Duration) {
// time is up, so change the state to LOW
PowerHead1State = LOW;
digitalWrite(PowerHead1, HIGH);
// and save the time when we made the change
previousPowerHead1Millis += PowerHead1Duration;
}
}
}
void updatePowerHead2State() {
if (PowerHead2State == LOW) {
// PowerHead2State = HIGH;
// digitalWrite(PowerHead2, LOW);
// }
if (currentMillis - previousPowerHead2Millis >= PowerHead2Interval) {
// time is up, so change the state to HIGH
PowerHead2State = HIGH;
digitalWrite(PowerHead2, LOW);
// and save the time when we made the change
previousPowerHead2Millis += PowerHead2Interval;
}
}
else {
if (currentMillis - previousPowerHead2Millis >= PowerHead2Duration) {
// time is up, so change the state to LOW
PowerHead2State = LOW;
digitalWrite(PowerHead2, HIGH);
// and save the time when we made the change
previousPowerHead2Millis += PowerHead2Duration;
}
}
}
void updatePowerHead3State() {
if (PowerHead3State == LOW) {
// PowerHead3State = HIGH;
// digitalWrite(PowerHead3, LOW);
// }
if (currentMillis - previousPowerHead3Millis >= PowerHead3Interval) {
// time is up, so change the state to HIGH
PowerHead3State = HIGH;
digitalWrite(PowerHead3, LOW);
// and save the time when we made the change
previousPowerHead3Millis += PowerHead3Interval;
}
}
else {
if (currentMillis - previousPowerHead3Millis >= PowerHead3Duration) {
// time is up, so change the state to LOW
PowerHead3State = LOW;
digitalWrite(PowerHead3, HIGH);
// and save the time when we made the change
previousPowerHead3Millis += PowerHead3Duration;
}
}
}
void updatePowerHead4State() {
if (PowerHead4State == LOW) {
// PowerHead4State = HIGH;
// digitalWrite(PowerHead4, LOW);
// }
if (currentMillis - previousPowerHead4Millis >= PowerHead4Interval) {
// time is up, so change the state to HIGH
PowerHead4State = HIGH;
digitalWrite(PowerHead4, LOW);
// and save the time when we made the change
previousPowerHead4Millis += PowerHead4Interval;
}
}
else {
if (currentMillis - previousPowerHead4Millis >= PowerHead4Duration) {
// time is up, so change the state to LOW
PowerHead4State = LOW;
digitalWrite(PowerHead4, HIGH);
// and save the time when we made the change
previousPowerHead4Millis += PowerHead4Duration;
}
}
}
void updateResFanState(){
if (ResFanState == LOW) {
ResFanState = HIGH;
digitalWrite(resFan, LOW);
}
// if (currentMillis - previousResFanMillis >= ResFanInterval) {
// // time is up, so change the state to HIGH
// ResFanState = HIGH;
// digitalWrite(resFan, LOW);
//
//
// // and save the time when we made the change
// previousResFanMillis += ResFanInterval;
// }
// }
// else {
//
// if (currentMillis - previousResFanMillis >= ResFanDuration) {
// // time is up, so change the state to LOW
// ResFanState = LOW;
// digitalWrite(resFan, HIGH);
//
// // and save the time when we made the change
// previousResFanMillis += ResFanDuration;
// }
// }
}
void updatePowerHeadState(){
if ((PowerHead1State == HIGH) || (PowerHead2State == HIGH) || (PowerHead3State == HIGH) || (PowerHead4State == HIGH)){
PowerHeadState = HIGH;
digitalWrite(powerHead, LOW);
} else {
PowerHeadState = LOW;
digitalWrite(powerHead, HIGH);
}
}
// if (currentMillis - previousPowerHeadMillis >= PowerHeadInterval) {
// // time is up, so change the state to HIGH
// PowerHeadState = HIGH;
// digitalWrite(powerHead, LOW);
//
// // and save the time when we made the change
// previousPowerHeadMillis += PowerHeadInterval;
// }
// }
// else {
//
// if (currentMillis - previousPowerHeadMillis >= PowerHeadDuration) {
// // time is up, so change the state to LOW
// PowerHeadState = LOW;
// digitalWrite(powerHead, HIGH);
//
//
//
// // and save the time when we made the change
// previousPowerHeadMillis += PowerHeadDuration;
// }
// }
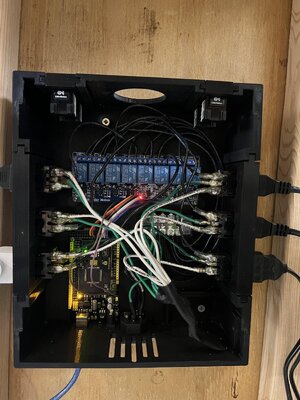